SwiftUI - 混合效果
SwiftUI 中的混合效果用于通过将两个或多个视图相互组合来创建各种 UI 设计。它是创建复杂且视觉上吸引人的设计的强大工具,也可以在 UI 设计中添加各种艺术效果。
使用此效果,我们可以混合任何组件,例如文本、图像、视图、容器等。此外,我们可以混合任意数量的图层,但最低要求是 2,即创建混合效果的基础图层和混合图层。
在本章中,我们将学习如何实现混合效果以及各种示例。
SwiftUI 中的".blendMode"修饰符
在 SwiftUI 中,我们可以借助预定义的 .blendMode 修饰符实现混合效果。此修饰符混合了另外两个视图以创建更美观的设计。它负责前景视图的颜色如何与背景视图的颜色混合。
语法
以下是语法 −
func blendMode(_blendMode:BlendMode) -> some View
此修饰符仅接受一个参数,即 blendMode。它表示我们要应用于给定视图的混合模式。
不同类型的模式
.blendMode 修饰符支持以下混合模式。这些模式可应用于任何包含文本、图像、形状等的视图。
它们分为各种类别,例如正常、变暗、变亮、对比度、反转、混合颜色成分、访问 poter-duff 模式等。
正常:这是 .blendMode 修饰符的默认模式。它不应用任何混合效果
变暗:这些模式用于通过混合源和目标来获得更暗的效果。
- darken
- multiply
- colorBurn
- plusDarker
Lighten: 这些模式用于对给定的视图应用亮化效果。
- lighten
- screen
- colorDodge
- plusLighter
Contrast: 这些模式用于在给定的视图上创建对比。
- overlay
- softLight
- hardLight
Invert: 这些模式根据目标颜色反转或取消源颜色。
- difference
- exclusion
Mixing Colors: 这些模式用于调整指定源和目标的颜色。
- hue
- saturation
- color
- luminosity
Poter-duff mode: 这些模式用于将不同类型的蒙版应用于指定 UIcomponenets 中的颜色 −
- sourceAtop
- destinationOver
- destinationOut
示例 1
以下 SwiftUI 程序在 colorBurn 模式下混合两个视图。
import SwiftUI struct ContentView: View { var body: some View { ZStack { Image("wallpaper") .resizable() .frame(width: 390, height: 300) .clipShape(Rectangle()) Rectangle() .fill(.blue) .frame(width: 390, height: 150) .blendMode(.colorBurn) } } } #Preview { ContentView() }
输出
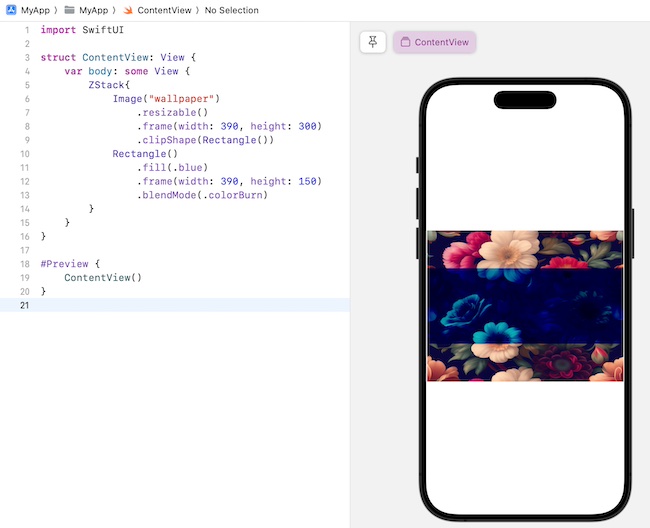
示例 2
以下 SwiftUI 程序在变暗模式下混合两个视图。
import SwiftUI struct ContentView: View { var body: some View { ZStack { Image("wallpaper") .resizable() .frame(width: 390, height: 300) .clipShape(Rectangle()) Rectangle() .fill(.blue) .frame(width: 390, height: 150) .blendMode(.darken) } } } #Preview { ContentView() }
输出
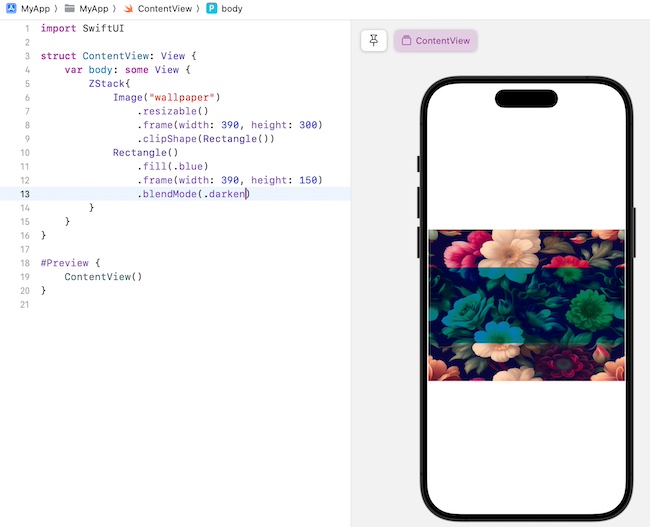
示例 3
以下 SwiftUI 程序在屏幕模式下混合两个视图。
import SwiftUI struct ContentView: View { var body: some View { ZStack { Image("wallpaper") .resizable() .frame(width: 390, height: 300) .clipShape(Rectangle()) Rectangle() .fill(.blue) .frame(width: 390, height: 150) .blendMode(.screen) } } } #Preview { ContentView() }
输出
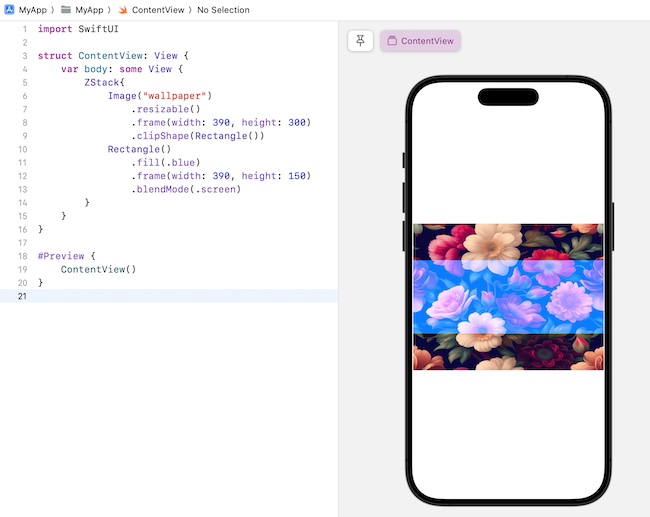
SwiftUI 中的多种混合模式
在 SwiftUI 中,我们不能同时使用多种混合模式修饰符,如果我们尝试这样做,则只有最近的 blendMode() 修饰符会采取行动,其余修饰符将被丢弃。
struct ContentView: View { var body: some View { ZStack { Rectangle() .fill(.blue) .frame(width: 390, height: 150) .blendMode(.darken) .blendMode(.destinationOut) .blendMode(.colorBurn) } } }
这里将执行 blendMode(.darken),而 .blendMode(.destinationOut) 和 .blendMode(.colorBurn) 将被丢弃。如果我们想使用多种混合模式,那么我们必须在不同的层中使用它们。
示例
以下 SwiftUI 程序使用多个 blendMode() 修饰符。
import SwiftUI struct ContentView: View { var body: some View { ZStack { Image("wallpaper") .resizable() .frame(width: 390, height: 300) .clipShape(Rectangle()) Rectangle() .fill(.blue) .frame(width: 390, height: 150) .blendMode(.colorBurn) Rectangle() .fill(.blue) .frame(width: 390, height: 150) .blendMode(.hardLight) } } } #Preview { ContentView() }
输出
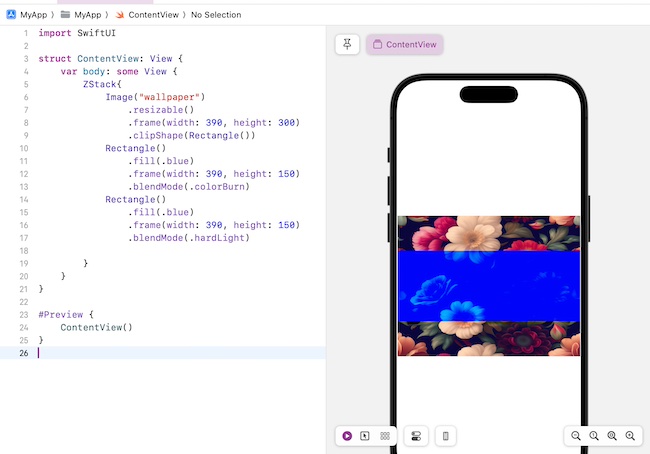