ReactJS - 日期选择器
React 通过第三方 UI 组件库提供表单组件。React 社区提供了大量的 UI / UX 组件,很难根据我们的需求选择合适的库。
Bootstrap UI 库是开发人员的热门选择之一,被广泛使用。 React Bootstrap (https://react-bootstrap.github.io/) 已将几乎所有 bootstrap UI 组件移植到 React 库,并且它对 date picker 组件也提供了最佳支持。
在本章中,让我们学习如何使用 react-bootstrap 库中的 date picker 组件。
什么是日期选择器?
日期选择器 允许开发人员轻松选择日期,而不必通过文本框输入正确的格式详细信息。HTML 输入元素具有类型属性,用于引用要输入到元素中的数据类型。其中一种类型是日期。在输入元素中设置类型将启用日期选择器。
<input type="date">
React bootstrap 提供 Form.Control 组件来创建各种输入元素。开发人员可以使用它来创建日期选择器控件。Form.Control 的一些有用属性如下 −
ref (ReactRef) − Ref 元素用于访问底层 DOM 节点
as (elementType) − 启用以指定除 *<input>* 之外的元素
disabled (boolean) −启用/禁用控制元素
htmlSize(数字) − 底层控制元素的大小
id(字符串) − 控制元素的 ID。如果未在此处指定,则使用父 *Form.Group* 组件的 *controlId*。
IsInValid(布尔值) − 启用/禁用与无效数据相关的样式
IsValid(布尔值) − 启用/禁用与有效数据相关的样式
plaintext(布尔值) − 启用/禁用输入并将其呈现为纯文本。与 *readonly* 属性一起使用
readOnly(布尔值) −启用/禁用控件的只读属性
size (sm | lg) − 输入元素的大小
type (string) − 要呈现的输入元素的类型
value (string | arrayOf | number) − 底层控件的值。由 *onChange* 事件操纵,初始值将默认为 *defaultValue* props
bsPrefix (string) − 用于自定义底层 CSS 类的前缀
onChange (boolean) − 触发 *onChange* 事件时要调用的回调函数
可以使用一个简单的日期控件组件,如下所示 −
<Form.Group className="mb-3" controlId="password"> <Form.Label>Date of birth</Form.Label> <Form.Control type="date" /> </Form.Group>
应用 Date picker 日期选择器 组件
首先,创建一个新的 React 应用程序并使用以下命令启动它。
create-react-app myapp cd myapp npm start
接下来,使用以下命令安装 bootstrap 库,
npm install --save bootstrap react-bootstrap
接下来,打开 App.css (src/App.css) 并删除所有 CSS 类。
// 删除 css 类
接下来,创建一个简单的日期组件 SimpleDatePicker (src/Components/SimpleDatePicker.js) 并呈现一个表单,如下所示 −
import { Form, Button } from 'react-bootstrap'; function SimpleDatePicker() { return ( <Form> <Form.Group className="mb-3" controlId="name"> <Form.Label>Name</Form.Label> <Form.Control type="name" placeholder="Enter your name" /> </Form.Group> <Form.Group className="mb-3" controlId="password"> <Form.Label>Date of birth</Form.Label> <Form.Control type="date" /> </Form.Group> <Button variant="primary" type="submit"> Submit </Button> </Form> ); } export default SimpleDatePicker;
这里我们有,
使用 Form.Control 和日期类型创建日期选择器控件。
使用 Form component 创建基本表单组件。
使用 Form.Group 对表单控件和标签进行分组。
接下来,打开 App 组件 (src/App.js),导入 bootstrap css 并呈现日期选择器,如下所示 −
import './App.css' import "bootstrap/dist/css/bootstrap.min.css"; import SimpleDatePicker from './Components/SimpleDatePicker' function App() { return ( <div className="container"> <div style={{ padding: "10px" }}> <div> <SimpleDatePicker /> </div> </div> </div> ); } export default App;
这里,
使用 import 语句导入 bootstrap 类
渲染我们新的 SimpleDatePicker 组件。
包含 App.css 样式
最后,在浏览器中打开应用程序并检查最终结果。日期选择器将呈现如下图所示 −
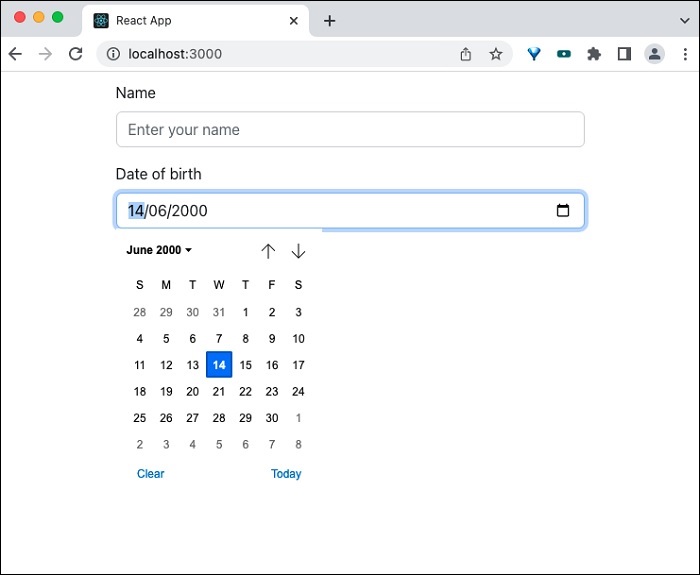
摘要
React Bootstrap 日期选择器组件提供了创建日期选择器表单控件所需的选项。