Ionic - Javascript 模态框
当激活 Ionic 模态框时,内容窗格将显示在常规内容之上。模态框基本上是具有更多功能的大型弹出窗口。默认情况下,模态框将覆盖整个屏幕,但可以根据需要进行优化。
使用模态框
在 Ionic 中实现模态框有两种方法。一种方法是添加单独的模板,另一种方法是将其添加到常规 HTML 文件的顶部,位于 script 标签内。我们需要做的第一件事是使用 angular 依赖注入将模态框连接到控制器。然后我们需要创建一个模态框。我们将传入范围并向模态框添加动画。
之后,我们将创建用于打开、关闭和销毁模态框的函数。最后两个函数位于我们可以编写代码的位置,如果模态框被隐藏或删除,这些代码将被触发。如果您不想在模式被移除或隐藏时触发任何功能,您可以删除最后两个函数。
控制器代码
.controller('MyController', function($scope, $ionicModal) { $ionicModal.fromTemplateUrl('my-modal.html', { scope: $scope, animation: 'slide-in-up' }).then(function(modal) { $scope.modal = modal; }); $scope.openModal = function() { $scope.modal.show(); }; $scope.closeModal = function() { $scope.modal.hide(); }; //使用完毕后清理模态框! $scope.$on('$destroy', function() { $scope.modal.remove(); }); // 隐藏模态框时执行操作 $scope.$on('modal.hidden', function() { // 执行操作 }); // 移除模态框时执行操作 $scope.$on('modal.removed', function() { // 执行操作 }); });
HTML 代码
<script id = "my-modal.html" type = "text/ng-template"> <ion-modal-view> <ion-header-bar> <h1 class = "title">Modal Title</h1> </ion-header-bar> <ion-content> <button class = "button icon icon-left ion-ios-close-outline" ng-click = "closeModal()">Close Modal</button> </ion-content> </ion-modal-view> </script>
我们在上一个示例中展示的方式是,当 script 标签用作某个现有 HTML 文件中模态框的容器时。
第二种方式是在 templates 文件夹中创建一个新的模板文件。我们将使用与上一个示例相同的代码,但我们将删除 script 标签,并且我们还需要更改控制器中的 fromTemplateUrl,以将模态框与新创建的模板连接起来。
控制器代码
.controller('MyController', function($scope, $ionicModal) { $ionicModal.fromTemplateUrl('templates/modal-template.html', { scope: $scope, animation: 'slide-in-up', }).then(function(modal) { $scope.modal = modal; }); $scope.openModal = function() { $scope.modal.show(); }; $scope.closeModal = function() { $scope.modal.hide(); }; //使用完毕后清理模态框! $scope.$on('$destroy', function() { $scope.modal.remove(); }); // 隐藏模态框时执行操作 $scope.$on('modal.hidden', function() { // 执行操作 }); // 移除模态框时执行操作 $scope.$on('modal.removed', function() { // 执行操作 }); });
HTML 代码
<ion-modal-view> <ion-header-bar> <h1 class = "title">Modal Title</h1> </ion-header-bar> <ion-content> <button class = "button icon icon-left ion-ios-close-outline" ng-click = "closeModal()">Close Modal</button> </ion-content> </ion-modal-view>
使用 Ionic 模式的第三种方法是内联插入 HTML。我们将使用 fromTemplate 函数代替 fromTemplateUrl。
控制器代码
.controller('MyController', function($scope, $ionicModal) { $scope.modal = $ionicModal.fromTemplate( '<ion-modal-view>' + ' <ion-header-bar>' + '<h1 class = "title">Modal Title</h1>' + '</ion-header-bar>' + '<ion-content>'+ '<button class = "button icon icon-left ion-ios-close-outline" ng-click = "closeModal()">Close Modal</button>' + '</ion-content>' + '</ion-modal-view>', { scope: $scope, animation: 'slide-in-up' }) $scope.openModal = function() { $scope.modal.show(); }; $scope.closeModal = function() { $scope.modal.hide(); }; //Cleanup the modal when we're done with it! $scope.$on('$destroy', function() { $scope.modal.remove(); }); // Execute action on hide modal $scope.$on('modal.hidden', function() { // Execute action }); // Execute action on remove modal $scope.$on('modal.removed', function() { // Execute action }); });
所有三个示例的效果都一样。我们将创建一个按钮来触发 $ionicModal.show() 以打开模态框。
HTML 代码
<button class = "button" ng-click = "openModal()"></button>
当我们打开模态框时,它将包含一个用于关闭它的按钮。我们在 HTML 模板中创建了这个按钮。
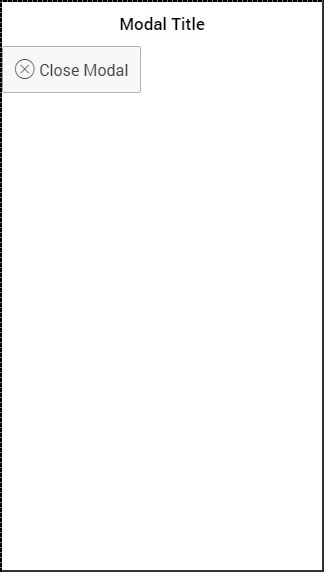
还有其他模态框优化选项。我们已经展示了如何使用 scope 和 animation。下表显示了其他选项。
选项 | 类型 | 详细信息 |
---|---|---|
focusFirstInput | boolean | 它将自动聚焦模态框的第一个输入。 |
backdropClickToClose | boolean | 当点击背景时,它将启用关闭模态框。默认值为 true。 |
hardwareBackButtonClose | boolean | 当单击硬件后退按钮时,将启用关闭模式。默认值为 true。 |