Mahotas - 软阈值
软阈值是指降低图像的噪声(去噪)以提高其质量。
它根据像素与阈值的接近程度为像素分配连续的值范围。这会导致前景和背景区域之间的逐渐过渡。
在软阈值中,阈值决定了去噪和图像保留之间的平衡。较高的阈值会导致更强的去噪,但会导致信息丢失。
相反,较低的阈值会保留更多信息,但会导致不必要的噪音。
Mahotas 中的软阈值
在 Mahotas 中,我们可以使用 thresholding.soft_threshold() 函数对图像应用软阈值。它根据相邻像素动态调整阈值,以增强噪声水平不均匀的图像。
通过使用动态调整,该函数按比例降低强度超过阈值的像素的强度,并将其分配给前景。
另一方面,如果像素的强度低于阈值,则将其分配给背景。
mahotas.thresholding.soft_threshold() 函数
mahotas.thresholding.soft_threshold() 函数以灰度图像作为输入,并返回已应用软阈值的图像。它通过将像素强度与提供的阈值进行比较来工作。
语法
以下是 mahotas − 中 soft_threshold() 函数的基本语法
mahotas.thresholding.soft_threshold(f, tval)
其中,
f − 是输入的灰度图像。
tval − 是阈值。
示例
在下面的示例中,我们使用 mh.thresholding.soft_threshold() 函数对灰度图像应用软阈值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # 加载图像 image = mh.imread('tree.tiff') # 将其转换为灰度 image = mh.colors.rgb2gray(image) # 设置阈值 tval = 150 # 在图像上应用软阈值 threshold_image = mh.thresholding.soft_threshold(image, tval) # 为子图创建图形和轴 fig, axis = mtplt.subplots(1, 2) # 显示原始图像 axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # 显示阈值图像 axes[1].imshow(threshold_image, cmap='gray') axes[1].set_title('Soft Threshold Image') axes[1].set_axis_off() # 调整间距子图 mtplt.tight_layout() # 显示图形 mtplt.show()
输出
以下是上述代码的输出 −
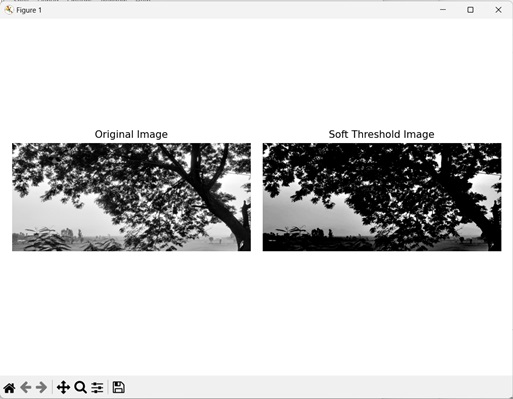
使用平均值的软阈值
我们可以使用图像上像素强度的平均值应用软阈值。平均值是指图像的平均强度。
它是通过将所有像素的强度值相加,然后除以总像素数来计算的。
在 mahotas 中,我们可以使用 numpy.mean() 函数找到图像所有像素的平均像素强度。然后可以将平均值传递给 mahotas.thresholding.soft_threshold() 函数的 tval 参数以生成软阈值图像。
这种应用软阈值的方法在去噪和图像质量之间保持了良好的平衡,因为阈值既不太高也不太低。
示例
以下示例显示了当阈值是像素强度的平均值时,在灰度图像上应用软阈值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # 加载图像 image = mh.imread('tree.tiff') # 将其转换为灰度 image = mh.colors.rgb2gray(image) # 设置平均阈值 tval = np.mean(image) # 在图像上应用软阈值 threshold_image = mh.thresholding.soft_threshold(image, tval) # 为子图创建图形和轴 fig, axis = mtplt.subplots(1, 2) # 显示原始图像 axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # 显示阈值图像 axes[1].imshow(threshold_image, cmap='gray') axes[1].set_title('Soft Threshold Image') axes[1].set_axis_off() #调整子图之间的间距 mtplt.tight_layout() # 显示图形 mtplt.show()
输出
上述代码的输出如下 −
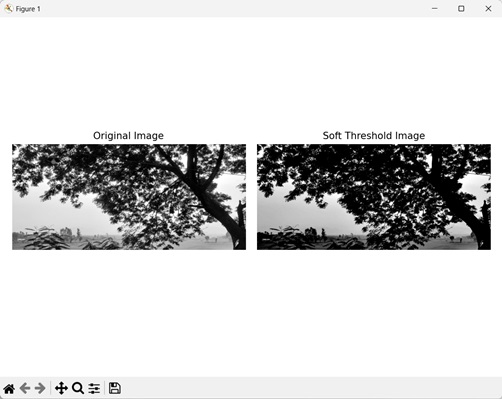
使用百分位值的软阈值
除了平均值,我们还可以使用百分位值对图像像素的强度应用软阈值。百分位是指给定百分比的数据低于该值的值;在图像处理中,它指的是图像中像素强度的分布。
例如,让我们将阈值百分位数设置为 85。这意味着只有强度大于图像中其他像素 85% 的像素才会被归类为前景,而其余像素将被归类为背景。
在 mahotas 中,我们可以使用 numpy.percentile() 函数根据像素强度的百分位数设置阈值。然后在 soft_thresholding() 函数中使用此值对图像应用软阈值。
示例
在此示例中,我们展示了当使用百分位数值找到阈值时如何应用软阈值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # 加载图像 image = mh.imread('tree.tiff') # 将其转换为灰度 image = mh.colors.rgb2gray(image) # 设置百分位阈值 tval = np.percentile(image, 85) # 在图像上应用软阈值 threshold_image = mh.thresholding.soft_threshold(image, tval) # 为子图创建图形和轴 fig, axis = mtplt.subplots(1, 2) # 显示原始图像 axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # 显示阈值图像 axes[1].imshow(threshold_image, cmap='gray') axes[1].set_title('Soft Threshold Image') axes[1].set_axis_off() # 调整子图之间的间距 mtplt.tight_layout() # 显示图形 mtplt.show()
输出
执行上述代码后,我们得到以下输出 −
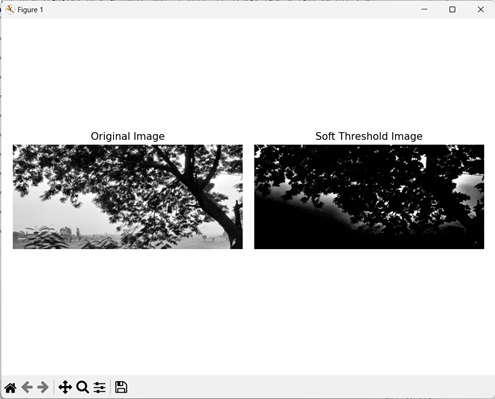