React Native - HTTP
在本章中,我们将向您展示如何使用 fetch 处理网络请求。
App.js
import React from 'react'; import HttpExample from './http_example.js' const App = () => { return ( <HttpExample /> ) } export default App
使用 Fetch
我们将使用 componentDidMount 生命周期方法在组件安装后立即从服务器加载数据。此函数将向服务器发送 GET 请求,返回 JSON 数据,将输出记录到控制台并更新我们的状态。
http_example.js
import React, { Component } from 'react' import { View, Text } from 'react-native' class HttpExample extends Component { state = { data: '' } componentDidMount = () => { fetch('https://jsonplaceholder.typicode.com/posts/1', { method: 'GET' }) .then((response) => response.json()) .then((responseJson) => { console.log(responseJson); this.setState({ data: responseJson }) }) .catch((error) => { console.error(error); }); } render() { return ( <View> <Text> {this.state.data.body} </Text> </View> ) } } export default HttpExample
输出
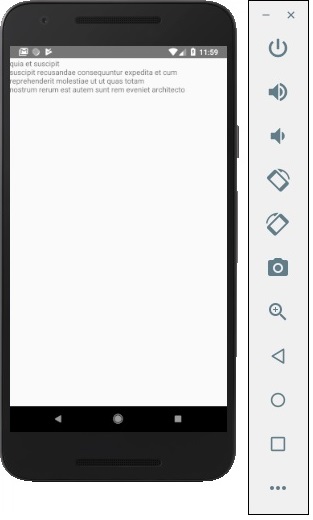