React Native - AsyncStorage
在本章中,我们将向您展示如何使用 AsyncStorage 保存数据。
步骤 1:演示
在此步骤中,我们将创建 App.js 文件。
import React from 'react' import AsyncStorageExample from './async_storage_example.js' const App = () => { return ( <AsyncStorageExample /> ) } export default App
步骤 2:逻辑
Name 的初始状态为空字符串。我们将在组件安装后从持久存储中更新它。
setName 将从我们的输入字段中获取文本,使用 AsyncStorage 保存它并更新状态。
async_storage_example.js
import React, { Component } from 'react' import { StatusBar } from 'react-native' import { AsyncStorage, Text, View, TextInput, StyleSheet } from 'react-native' class AsyncStorageExample extends Component { state = { 'name': '' } componentDidMount = () => AsyncStorage.getItem('name').then((value) => this.setState({ 'name': value })) setName = (value) => { AsyncStorage.setItem('name', value); this.setState({ 'name': value }); } render() { return ( <View style = {styles.container}> <TextInput style = {styles.textInput} autoCapitalize = 'none' onChangeText = {this.setName}/> <Text> {this.state.name} </Text> </View> ) } } export default AsyncStorageExample const styles = StyleSheet.create ({ container: { flex: 1, alignItems: 'center', marginTop: 50 }, textInput: { margin: 5, height: 100, borderWidth: 1, backgroundColor: '#7685ed' } })
当我们运行应用程序时,我们可以通过在输入字段中输入内容来更新文本。
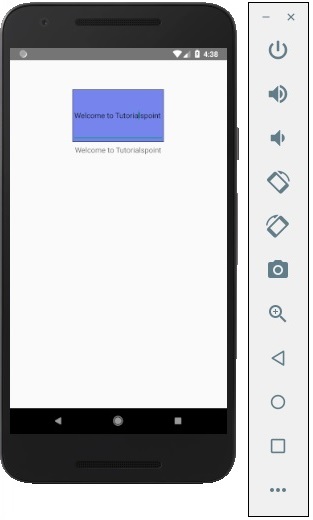