C - if-else 语句
if-else 语句是 C 语言中常用的决策语句之一。当条件不满足时,if-else 语句提供了一条替代路径。
else 关键字可帮助您在 if 语句中的布尔表达式为假时提供替代操作方案。else 关键字的使用是可选的;是否使用它由您决定。
if-else 语句的语法
if-else 子句的语法如下:
if (Boolean expr){ Expression; . . . } else{ Expression; . . . }
C 编译器会评估条件,如果条件为真,则执行 if 语句 后面的一条语句或一组语句。
如果编程逻辑需要计算机在条件为假时执行其他指令,则这些指令会被放在 else 子句中。
if 语句后面跟着一个可选的 else 语句,该语句在布尔表达式为假时执行。
if-else 语句流程图
以下流程图展示了 if-else 子句在 C 语言中的工作方式 -
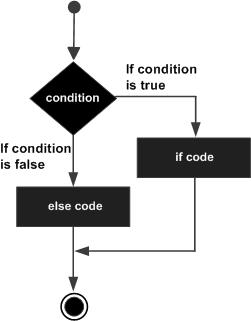
请注意,如果要执行多个语句,则 if 和 else 子句中的花括号是必需的。例如,在下面的代码中,我们不需要花括号。
if (marks<50) printf("Result: Fail "); else printf("Result: Pass ");
但是,当 if 或 else 部分有多个语句时,您需要告诉编译器它们需要被视为复合语句。
C 语言 if-else 语句示例
示例:使用 if-else 语句计算税款
在下面的代码中,计算了员工收入的税率。如果收入低于 10,000,则适用 10% 的税率。对于超过 10,000 的收入,超出部分将按 15% 征收。
#include <stdio.h> int main() { int income = 5000; float tax; printf("Income: %d ", income); if (income<10000){ tax = (float)(income * 10 / 100); printf("tax: %f ", tax); } else { tax= (float)(1000 + (income-10000) * 15 / 100); printf("tax: %f", tax); } }
输出
运行代码并检查其输出 −
Income: 5000 tax: 500.000000
将收入变量设置为 15000,然后再次运行程序。
Income: 15000 tax: 1750.000000
示例:使用 if-else 语句检查数字
以下程序检查 char 变量存储的是数字还是非数字字符。
#include <stdio.h> int main() { char ch='7'; if (ch>=48 && ch<=57){ printf("The character is a digit."); } else{ printf("The character is not a digit."); } return 0; }
输出
运行代码并检查其输出 −
The character is a digit.
将任何其他字符(例如"*")赋值给"ch",并查看结果。
The character is not a digit.
示例:不带花括号的 if-else 语句
考虑以下代码。如果金额大于 100,则按 10% 计算折扣,否则不计算折扣。
#include <stdio.h> int main() { int amount = 50; float discount; printf("Amount: %d ", amount); if (amount >= 100) discount = amount * 10 / 100; printf("Discount: %f ", discount); else printf("Discount not applicable "); return 0; }
输出
程序在编译过程中显示以下错误 -
error: 'else' without a previous 'if'
编译器将执行 if 子句后的第一个语句,并假设由于下一个语句不是 else(它本身就是可选的),因此后续的 printf() 语句是无条件的。然而,下一个 else 语句并未连接到任何 if 语句,因此会报错。
示例:不带花括号的 if-else 语句
也请考虑以下代码 -
#include <stdio.h> int main() { int amount = 50; float discount, nett; printf("Amount: %d ", amount); if (amount<100) printf("Discount not applicable "); else printf("Discount applicable"); discount = amount*10/100; nett = amount - discount; printf("Discount: %f Net payable: %f", discount, nett); return 0; }
输出
代码没有给出任何编译器错误,但给出了不正确的输出 -
Amount: 50 Discount not applicable Discount: 5.000000 Net payable: 45.000000
它会产生错误的输出,因为编译器假设 else 子句中只有一个语句,其余语句都是无条件的。
以上两个代码示例强调了这样一个事实:当 if 或 else else 语句中有多个语句时,必须将它们放在大括号中。
为了安全起见,即使对于单个语句,也最好使用大括号。事实上,它提高了代码的可读性。
上述问题的正确解决方案如下所示 -
if (amount >= 100){ discount = amount * 10 / 100; printf("Discount: %f ", discount); } else { printf("Discount not applicable "); }
C 语言中的 else-if 语句
C 语言也允许在程序中使用 else-if 语句。让我们看看哪些情况下可能需要使用 else-if 子句。
假设您遇到如下情况。如果某个条件成立,则运行其后的给定代码块。如果条件不成立,则运行下一个代码块。但是,如果以上条件都不成立,并且所有其他条件都失败,则最终运行另一个代码块。在这种情况下,您可以使用 else-if 子句。
else-if 语句的语法
以下是 else-if 子句的语法 -
if (condition){ // 如果条件为真, // 则运行此代码 } else if(another_condition){ // 如果上述条件为假, // 且此条件为真, // 则运行此代码块中的代码 } else{ // 如果上述两个条件均为假, // 则运行此代码 }
else-if 语句示例
请看以下示例 -
#include <stdio.h> int main(void) { int age = 15; if (age < 18) { printf("You need to be over 18 years old to continue "); }else if (age < 21) { printf("You need to be over 21 "); } else { printf("You are over 18 and older than 21 so you can continue "); } }
输出
运行代码并检查其输出 −
You need to be over 18 years old to continue
现在,为变量"age"提供不同的值,然后再次运行代码。如果提供的值小于 18,您将得到不同的输出。