XAML - TimePicker
TimePicker 是一个允许用户选择时间值的控件。TimePicker 类的层次继承如下 −
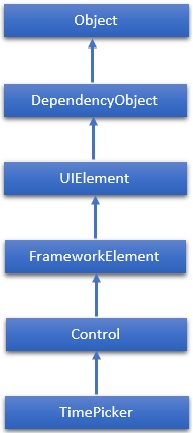
属性
Sr.No. | 属性 &描述 |
---|---|
1 | ClockIdentifier 获取或设置要使用的时钟系统。 |
2 | ClockIdentifierProperty 获取 ClockIdentifier 依赖项属性的标识符。 |
3 | Header 获取或设置控件标题的内容。 |
4 | HeaderProperty 标识 Header 依赖项属性。 |
5 | HeaderTemplate 获取或设置用于显示控件标题内容的 DataTemplate。 |
6 | HeaderTemplateProperty 标识 HeaderTemplate 依赖项属性。 |
7 | MinuteIncrement 获取或设置指示分钟选择器中显示的时间增量的值。例如,15 指定 TimePicker 分钟控件仅显示选项 00、15、30、45。 |
8 | MinuteIncrementProperty 获取 MinuteIncrement 依赖项属性的标识符。 |
9 | Time 获取或设置时间选择器中当前设置的时间。 |
10 | TimeProperty 获取 Time 依赖项属性的标识符。 |
事件
Sr.No. | 事件和说明 |
---|---|
1 | ManipulationCompleted 当对 UIElement 的操作完成时发生。 (从 UIElement 继承) |
2 | ManipulationDelta 当输入设备在操作过程中改变位置时发生。 (从 UIElement 继承) |
3 | ManipulationInertiaStarting 当输入设备在操作过程中与 UIElement 对象失去联系并且开始惯性时发生。 (从 UIElement 继承) |
4 | ManipulationStarted 当输入设备开始对 UIElement 进行操作时发生。 (从 UIElement 继承) |
5 | ManipulationStarting 首次创建操作处理器时发生。 (从 UIElement 继承) |
6 | TimeChanged 时间值改变时发生。 |
方法
Sr.No. | 方法 &描述 |
---|---|
1 | OnManipulationCompleted 在 ManipulationCompleted 事件发生之前调用。(从控件继承) |
2 | OnManipulationDelta 在 ManipulationDelta 事件发生之前调用。(从控件继承) |
3 | OnManipulationInertiaStarting 在 ManipulationInertiaStarting 事件发生之前调用。(从控件继承) |
4 | OnManipulationStarted 在 ManipulationStarted 事件发生之前调用。(从控件继承) |
5 | OnManipulationStarting 在 ManipulationStarting 事件发生之前调用。(从控件继承) |
示例
以下示例显示了 TimePicker 在 XAML 应用程序中的用法。以下是创建和初始化 TimePicker 及其一些属性的 XAML 代码。
<Page x:Class = "XAMLTimePicker.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local = "using:XAMLTimePicker" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d"> <Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}"> <StackPanel Orientation = "Horizontal" Height = "60" Margin = "46,67,-46,641"> <TimePicker x:Name = "arrivalTimePicker" Header = "Arrival Time" Margin = "0,1"/> <Button Content = "Submit" Click = "SubmitButton_Click" Margin = "5,0,0,-2" VerticalAlignment = "Bottom"/> <TextBlock x:Name = "Control1Output" FontSize = "24"/> </StackPanel> </Grid> </Page>
以下是 C# 中的点击事件实现 −
using System; using Windows.UI.Xaml; using Windows.UI.Xaml.Controls; namespace XAMLTimePicker { public sealed partial class MainPage : Page { public MainPage() { this.InitializeComponent(); } private void SubmitButton_Click(object sender, RoutedEventArgs e) { if (VerifyTimeIsAvailable(arrivalTimePicker.Time) == true) { Control1Output.Text = string.Format("Thank you. Your appointment is set for {0}.", arrivalTimePicker.Time.ToString()); } else { Control1Output.Text = "Sorry, we're only open from 8AM to 5PM."; } } private bool VerifyTimeIsAvailable(TimeSpan time) { // Set open (8AM) and close (5PM) times. TimeSpan openTime = new TimeSpan(8, 0, 0); TimeSpan closeTime = new TimeSpan(17, 0, 0); if (time >= openTime && time < closeTime) { return true; // Open } return false; // Closed } } }
当您编译并执行上述代码时,它将显示以下输出。当选择的时间在上午 8 点到下午 5 点之间时,它将显示以下消息 −
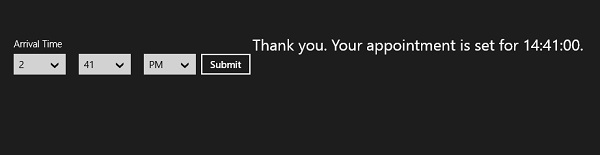
否则,将显示以下消息 −
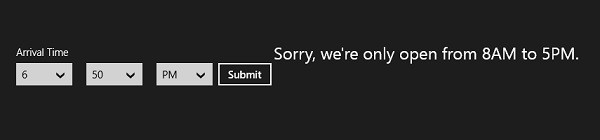
我们建议您执行上述示例代码并尝试一些其他属性和事件。