MFC - 字符串
字符串是表示字符序列的对象。 C 样式字符串起源于 C 语言,并继续在 C++ 中得到支持。
该字符串实际上是一个一维字符数组,以空字符"\0"结尾。
以 null 结尾的字符串包含构成该字符串的字符,后跟一个 null。
这是字符数组的简单示例。
char word[12] = { 'H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd', '\0' };
以下是另一种表示方式。
char word[] = "Hello, World";
Microsoft 基础类 (MFC) 库提供了一个名为 CString 的字符串操作类。 以下是 CString 的一些重要特性。
CString 没有基类。
CString 对象由可变长度的字符序列组成。
CString 使用与 Basic 类似的语法提供函数和运算符。
连接和比较运算符以及简化的内存管理使 CString 对象比普通字符数组更易于使用。
这是 CString 的构造函数。
序号 | 方法及描述 |
---|---|
1 | CString 以多种方式构造 CString 对象 |
这是数组方法的列表 −
序号 | 方法及描述 |
---|---|
1 | GetLength 返回 CString 对象中的字符数。 |
2 | IsEmpty 测试 CString 对象是否不包含字符。 |
3 | Empty 强制字符串长度为 0。 |
4 | GetAt 返回指定位置的字符。 |
5 | SetAt 在指定位置设置一个字符。 |
这里是比较方法的列表 −
序号 | 方法及描述 |
---|---|
1 | Compare 比较两个字符串(区分大小写)。 |
2 | CompareNoCase 比较两个字符串(不区分大小写)。 |
这是提取方法的列表 −
序号 | 方法及描述 |
---|---|
1 | Mid 提取字符串的中间部分(如 Basic MID$ 函数)。 |
2 | Left 提取字符串的左侧部分(如基本 LEFT$ 函数)。 |
3 | Right 提取字符串的右侧部分(如基本 RIGHT$ 函数)。 |
4 | SpanIncluding 从字符串中提取给定字符集中的字符。 |
5 | SpanExcluding 从字符串中提取不属于给定字符集中的字符。 |
这里是转换方法的列表。
序号 | 方法及描述 |
---|---|
1 | MakeUpper 将此字符串中的所有字符转换为大写字符。 |
2 | MakeLower 将此字符串中的所有字符转换为小写字符。 |
3 | MakeReverse 反转该字符串中的字符。 |
4 | Format 像 sprintf 一样格式化字符串。 |
5 | TrimLeft 修剪字符串中的前导空白字符。 |
6 | TrimRight 修剪字符串中的尾随空白字符。 |
这里是搜索方法的列表。
序号 | 方法及描述 |
---|---|
1 | Find 在较大字符串中查找字符或子字符串。 |
2 | ReverseFind 在较大字符串中查找字符; 从最后开始。 |
3 | FindOneOf 从集合中查找第一个匹配的字符。 |
这里是缓冲区访问方法的列表。
序号 | 方法及描述 |
---|---|
1 | GetBuffer 返回指向 CString 中字符的指针。 |
2 | GetBufferSetLength 返回指向 CString 中字符的指针,截断为指定长度。 |
3 | ReleaseBuffer 释放对 GetBuffer 返回的缓冲区的控件 |
4 | FreeExtra 通过释放先前分配给字符串的任何额外内存来消除此字符串对象的任何开销。 |
5 | LockBuffer 禁用引用计数并保护缓冲区中的字符串。 |
6 | UnlockBuffer 启用引用计数并释放缓冲区中的字符串。 |
这是 Windows 特定方法的列表。
序号 | 方法及描述 |
---|---|
1 | AllocSysString 从 CString 数据分配 BSTR。 |
2 | SetSysString 使用 CString 对象中的数据设置现有 BSTR 对象。 |
3 | 加载字符串 从 Windows CE 资源加载现有的 CString 对象。 |
以下是对 CString 对象的不同操作 −
创建字符串
您可以通过使用字符串文字或创建 CString 类的实例来创建字符串。
BOOL CMFCStringDemoDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialog SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon CString string1 = _T("This is a string1"); CString string2("This is a string2"); m_strText.Append(string1 + L"\n"); m_strText.Append(string2); UpdateData(FALSE); return TRUE; // return TRUE unless you set the focus to a control }
编译并执行上述代码后,您将看到以下输出。
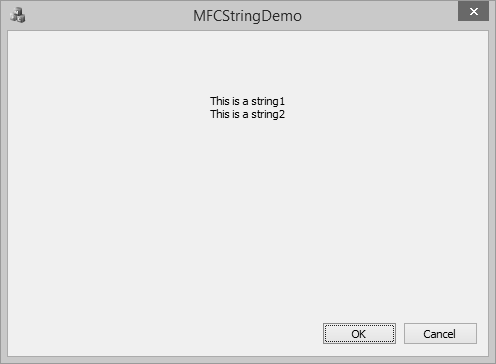
空字符串
您可以通过使用空字符串文字或使用 CString::Empty() 方法来创建空字符串。 您还可以使用布尔属性 isEmpty 检查字符串是否为空。
BOOL CMFCStringDemoDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialog SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon CString string1 = _T(""); CString string2; string2.Empty(); if(string1.IsEmpty()) m_strText.Append(L"String1 is empty\n"); else m_strText.Append(string1 + L"\n"); if(string2.IsEmpty()) m_strText.Append(L"String2 is empty"); else m_strText.Append(string2); UpdateData(FALSE); return TRUE; // return TRUE unless you set the focus to a control }
编译并执行上述代码后,您将看到以下输出。
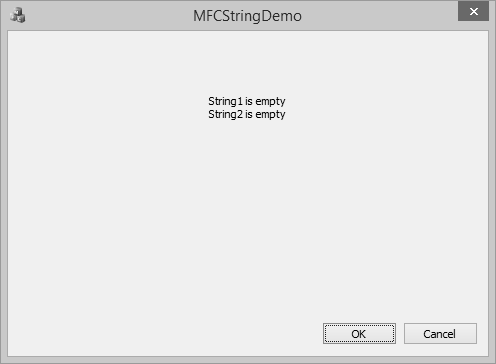
字符串连接
要连接两个或多个字符串,可以使用 + 运算符连接两个字符串或使用 CString::Append() 方法。
BOOL CMFCStringDemoDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialog SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon //To concatenate two CString objects CString s1 = _T("This "); // Cascading concatenation s1 += _T("is a "); CString s2 = _T("test"); CString message = s1; message.Append(_T("big ") + s2); // Message contains "This is a big test". m_strText = L"message: " + message; UpdateData(FALSE); return TRUE; // return TRUE unless you set the focus to a control }
编译并执行上述代码后,您将看到以下输出。
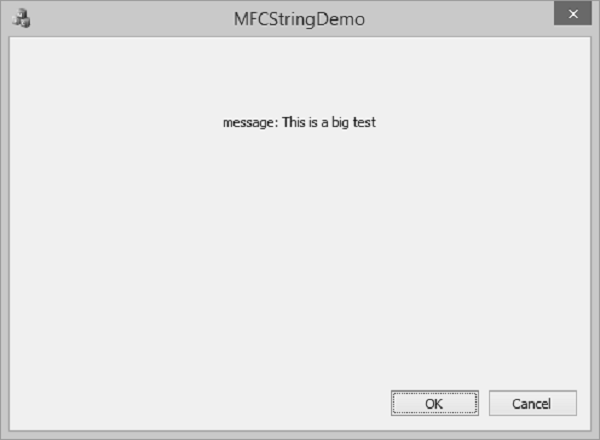
字符串长度
要查找字符串的长度,可以使用 CString::GetLength() 方法,该方法返回 CString 对象中的字符数。
BOOL CMFCStringDemoDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialog SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon CString string1 = _T("This is string 1"); int length = string1.GetLength(); CString strLen; strLen.Format(L"\nString1 contains %d characters", length); m_strText = string1 + strLen; UpdateData(FALSE); return TRUE; // return TRUE unless you set the focus to a control }
编译并执行上述代码后,您将看到以下输出。
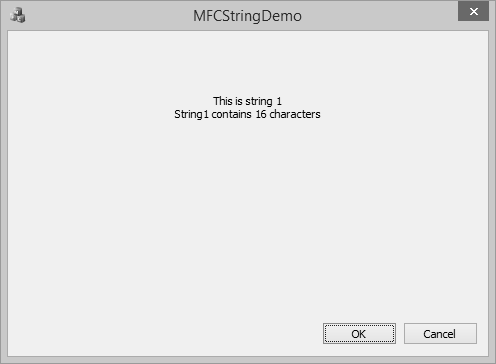
字符串比较
要比较两个字符串变量,可以使用 == 运算符
BOOL CMFCStringDemoDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialog SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon CString string1 = _T("Hello"); CString string2 = _T("World"); CString string3 = _T("MFC Tutorial"); CString string4 = _T("MFC Tutorial"); if (string1 == string2) m_strText = "string1 and string1 are same\n"; else m_strText = "string1 and string1 are not same\n"; if (string3 == string4) m_strText += "string3 and string4 are same"; else m_strText += "string3 and string4 are not same"; UpdateData(FALSE); return TRUE; // return TRUE unless you set the focus to a control }
编译并执行上述代码后,您将看到以下输出。
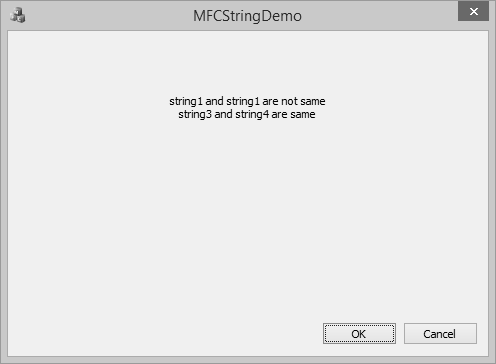