Three.js - Spotlight 聚光灯
这是另一种以圆锥体形状从特定方向发出的光。
distance − 光的最大范围。默认值为 0(无限制)。
angle − 光从其方向散射的最大角度,其上限为 Math.PI/2。
penumbra − 聚光灯锥体因半影而衰减的百分比。取值介于 0 和 1 之间。默认值为 0。
decay −光线随光线距离变暗的程度。
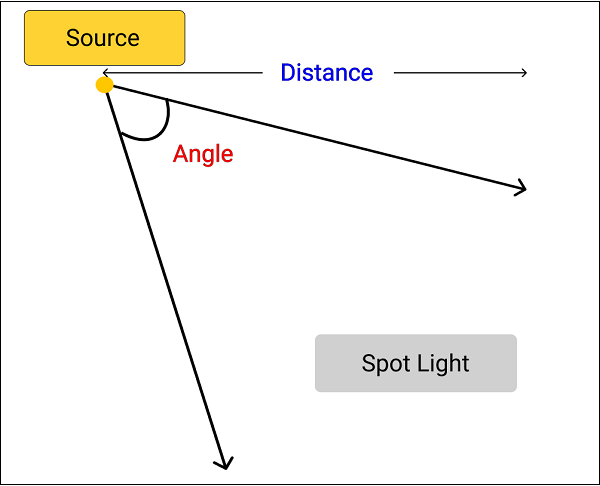
const light = new THREE.SpotLight(color, intense) light.position.set(1, 10, 10) light.castShadow = true light.shadow.camera.near = 5 light.shadow.camera.far = 400 light.shadow.camera.fov = 30
shadow.camera.near、shadow.camera.far 和 shadow.camera.fov 属性定义阴影可以出现的区域。
查看以下示例。
示例
spotlight.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - SpotLight</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="container"></div> <script type="module"> // 在 Three.js 中添加聚光灯 // 您可以使用 GUI 控制光的属性 // 您可以在此示例中看到位置和光锥 // GUI const gui = new dat.GUI() // 尺寸 const width = window.innerWidth const height = window.innerHeight // 场景 const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) console.log(scene.children) // 相机 const camera = new THREE.PerspectiveCamera(60, width / height, 0.1, 1000) camera.position.set(0, 0, 10) const camFolder = gui.addFolder('Camera') camFolder.add(camera.position, 'z', 10, 80, 1) camFolder.open() // lights const ambientLight = new THREE.AmbientLight(0xffffff, 0.5) scene.add(ambientLight) const light = new THREE.SpotLight() light.position.set(0, 5, 0) // 对于阴影 light.castShadow = true light.shadow.mapSize.width = 1024 light.shadow.mapSize.height = 1024 light.shadow.camera.near = 0.5 light.shadow.camera.far = 100 scene.add(light) const helper = new THREE.SpotLightHelper(light) scene.add(helper) // 灯光控制 const lightColor = { color: light.color.getHex() } const lightFolder = gui.addFolder('Light') lightFolder.addColor(lightColor, 'color').onChange(() => { light.color.set(lightColor.color) }) lightFolder.add(light, 'intensity', 0, 1, 0.01) lightFolder.open() const spotLightFolder = gui.addFolder('SpotLight') spotLightFolder.add(light, 'distance', 0, 100, 0.01) spotLightFolder.add(light, 'decay', 0, 4, 0.1) spotLightFolder.add(light, 'angle', 0, 1, 0.1) spotLightFolder.add(light, 'penumbra', 0, 1, 0.1) spotLightFolder.add(light.position, 'x', -50, 50, 1) spotLightFolder.add(light.position, 'y', -50, 50, 1) spotLightFolder.add(light.position, 'z', -50, 50, 1) spotLightFolder.open() // plane const planeGeometry = new THREE.PlaneGeometry(100, 100) const plane = new THREE.Mesh(planeGeometry, new THREE.MeshPhongMateria l({ color: 0xffffff })) plane.rotateX(-Math.PI / 2) plane.position.y = -1.75 plane.receiveShadow = true scene.add(plane) // cube const geometry = new THREE.BoxGeometry(2, 2, 2) const material = new THREE.MeshStandardMaterial({ color: 0x87ceeb }) const materialFolder = gui.addFolder('Material') materialFolder.add(material, 'wireframe') materialFolder.open() const cube = new THREE.Mesh(geometry, material) cube.position.set(0, 0.5, 0) cube.castShadow = true cube.receiveShadow = true scene.add(cube) // 响应性 window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // 渲染器 const renderer = new THREE.WebGL1Renderer() renderer.setSize(window.innerWidth, window.innerHeight) renderer.shadowMap.enabled = true renderer.shadowMap.type = THREE.PCFSoftShadowMap renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // 动画 function animate() { requestAnimationFrame(animate) cube.rotation.x += 0.005 cube.rotation.y += 0.01 renderer.render(scene, camera) } // 渲染场景 const container = document.querySelector('#container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
输出
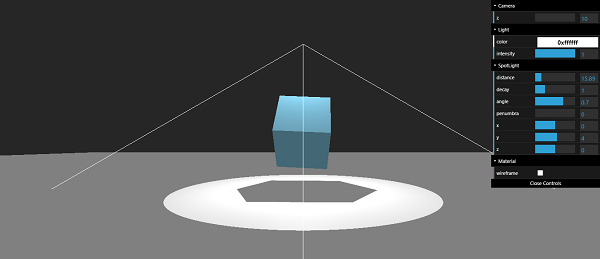
threejs_lights_and_shadows.html