Laravel - Facades
Facades 为应用程序服务容器中可用的类提供静态接口。Laravel facades 充当服务容器中底层类的静态代理,提供简洁、富有表现力的语法优势,同时比传统静态方法保持更高的可测试性和灵活性。
如何创建 Facade
以下是在 Laravel 中创建 Facade 的步骤 −
步骤 1 − 创建 PHP 类文件。
步骤 2 − 将该类绑定到服务提供商。
步骤 3 −将该 ServiceProvider 注册到
Config\app.php 作为提供程序。
步骤 4 − 创建该类扩展的类
lluminate\Support\Facades\Facade。
步骤 5 − 将第 4 点注册到 Config\app.php 作为别名。
Facade 类参考
Laravel 附带许多 Facade。下表显示内置的 Facade 类参考 −
Facade | 类 | 服务容器绑定 |
---|---|---|
App | Illuminate\Foundation\Application | app |
Artisan | Illuminate\Contracts\Console\Kernel | artisan |
Auth | Illuminate\Auth\AuthManager | auth |
Auth (Instance) | Illuminate\Auth\Guard | |
Blade | Illuminate\View\Compilers\BladeCompiler | blade.compiler |
Bus | Illuminate\Contracts\Bus\Dispatcher | |
Cache | Illuminate\Cache\Repository | cache |
Config | Illuminate\Config\Repository | config |
Cookie | Illuminate\Cookie\CookieJar | cookie |
Crypt | Illuminate\Encryption\Encrypter | encrypter |
DB | Illuminate\Database\DatabaseManager | db |
DB (Instance) | Illuminate\Database\Connection | |
Event | Illuminate\Events\Dispatcher | events |
File | Illuminate\Filesystem\Filesystem | files |
Gate | Illuminate\Contracts\Auth\Access\Gate | |
Hash | Illuminate\Contracts\Hashing\Hasher | hash |
Input | Illuminate\Http\Request | request |
Lang | Illuminate\Translation\Translator | translator |
Log | Illuminate\Log\Writer | log |
Illuminate\Mail\Mailer | mailer | |
Password | Illuminate\Auth\Passwords\PasswordBroker | auth.password |
Queue | Illuminate\Queue\QueueManager | queue |
Queue (Instance) | Illuminate\Queue\QueueInterface | |
Queue (Base Class) | Illuminate\Queue\Queue | |
Redirect | Illuminate\Routing\Redirector | redirect |
Redis | Illuminate\Redis\Database | redis |
Request | Illuminate\Http\Request | request |
Response | Illuminate\Contracts\Routing\ResponseFactory | |
Route | Illuminate\Routing\Router | router |
Schema | Illuminate\Database\Schema\Blueprint | |
Session | Illuminate\Session\SessionManager | session |
Session (Instance) | Illuminate\Session\Store | |
Storage | Illuminate\Contracts\Filesystem\Factory | filesystem |
URL | Illuminate\Routing\UrlGenerator | url |
Validator | Illuminate\Validation\Factory | validator |
Validator (Instance) | Illuminate\Validation\Validator | |
View | Illuminate\View\Factory | view |
View (Instance) | Illuminate\View\View |
示例
步骤 1 − 通过执行以下命令创建一个名为 TestFacadesServiceProvider 的服务提供程序。
php artisan make:provider TestFacadesServiceProvider
步骤 2 − 执行成功后,您将收到以下输出 −
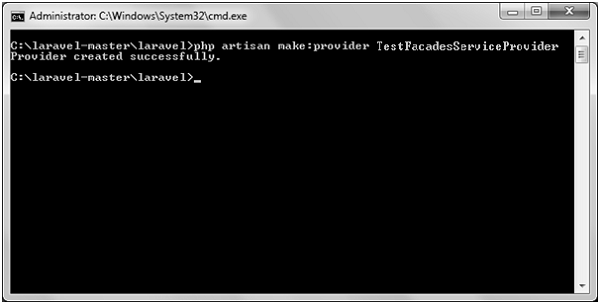
步骤 3 − 在 App/Test 中创建一个名为 TestFacades.php 的类。
App/Test/TestFacades.php
<?php namespace App\Test; class TestFacades{ public function testingFacades() { echo "Testing the Facades in Laravel."; } } ?>
步骤 4 − 在 "App/Test/Facades" 处创建一个名为 "TestFacades.php" 的 Facade 类。
App/Test/Facades/TestFacades.php
<?php namespace app\Test\Facades; use Illuminate\Support\Facades\Facade; class TestFacades extends Facade { protected static function getFacadeAccessor() { return 'test'; } }
步骤 5 − 在 App/Test/Facades 处创建一个名为 TestFacadesServiceProviders.php 的 Facade 类。
App/Providers/TestFacadesServiceProviders.php
<?php namespace App\Providers; use App; use Illuminate\Support\ServiceProvider; class TestFacadesServiceProvider extends ServiceProvider { public function boot() { // } public function register() { App::bind('test',function() { return new \App\Test\TestFacades; }); } }
步骤 6 − 在文件 config/app.php 中添加服务提供者,如下图所示。
config/app.php
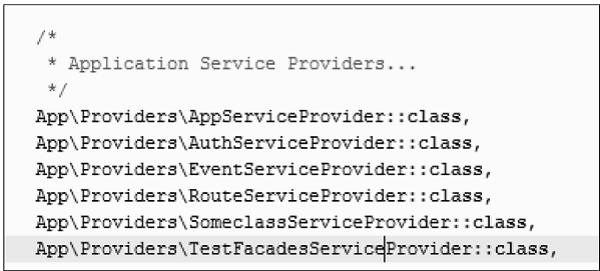
步骤 7 − 在文件 config/app.php 中添加别名,如下图所示。
config/app.php
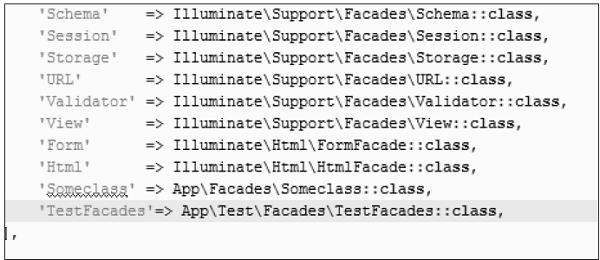
步骤 8 −在 app/Http/routes.php 中添加以下几行。
app/Http/routes.php
Route::get('/facadeex', function() { return TestFacades::testingFacades(); });
步骤 9 − 访问以下 URL 以测试 Facade。
http://localhost:8000/facadeex
步骤 10 − 访问 URL 后,您将收到以下输出 −
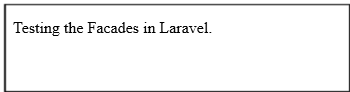