Java RMI - 数据库应用程序
在上一章中,我们创建了一个示例 RMI 应用程序,其中客户端调用显示 GUI 窗口 (JavaFX) 的方法。
在本章中,我们将通过一个示例来了解客户端程序如何检索驻留在服务器上的 MySQL 数据库中的表的记录。
假设数据库details中有一个名为student_data的表,如下所示。
+----+--------+--------+------------+---------------------+ | ID | NAME | BRANCH | PERCENTAGE | EMAIL | +----+--------+--------+------------+---------------------+ | 1 | Ram | IT | 85 | ram123@gmail.com | | 2 | Rahim | EEE | 95 | rahim123@gmail.com | | 3 | Robert | ECE | 90 | robert123@gmail.com | +----+--------+--------+------------+---------------------+
假设用户名为myuser,密码为password。
创建学生班级
使用 setter 和 getter 方法创建一个 Student 类,如下所示。
public class Student implements java.io.Serializable { private int id, percent; private String name, branch, email; public int getId() { return id; } public String getName() { return name; } public String getBranch() { return branch; } public int getPercent() { return percent; } public String getEmail() { return email; } public void setID(int id) { this.id = id; } public void setName(String name) { this.name = name; } public void setBranch(String branch) { this.branch = branch; } public void setPercent(int percent) { this.percent = percent; } public void setEmail(String email) { this.email = email; } }
定义远程接口
定义远程接口。 在这里,我们定义了一个名为 Hello 的远程接口,其中包含一个名为 getStudents () 的方法。 此方法返回一个列表,其中包含 Student 类的对象。
import java.rmi.Remote; import java.rmi.RemoteException; import java.util.*; // Creating Remote interface for our application public interface Hello extends Remote { public List<Student> getStudents() throws Exception; }
开发实现类
创建一个类并实现上面创建的接口。
这里我们正在实现远程接口的getStudents()方法。 当您调用此方法时,它会检索名为 student_data 的表的记录。 使用其 setter 方法将这些值设置到 Student 类,将其添加到列表对象并返回该列表。
import java.sql.*; import java.util.*; // Implementing the remote interface public class ImplExample implements Hello { // Implementing the interface method public List<Student> getStudents() throws Exception { List<Student> list = new ArrayList<Student>(); // JDBC driver name and database URL String JDBC_DRIVER = "com.mysql.jdbc.Driver"; String DB_URL = "jdbc:mysql://localhost:3306/details"; // Database credentials String USER = "myuser"; String PASS = "password"; Connection conn = null; Statement stmt = null; //Register JDBC driver Class.forName("com.mysql.jdbc.Driver"); //Open a connection System.out.println("Connecting to a selected database..."); conn = DriverManager.getConnection(DB_URL, USER, PASS); System.out.println("Connected database successfully..."); //Execute a query System.out.println("Creating statement..."); stmt = conn.createStatement(); String sql = "SELECT * FROM student_data"; ResultSet rs = stmt.executeQuery(sql); //Extract data from result set while(rs.next()) { // Retrieve by column name int id = rs.getInt("id"); String name = rs.getString("name"); String branch = rs.getString("branch"); int percent = rs.getInt("percentage"); String email = rs.getString("email"); // Setting the values Student student = new Student(); student.setID(id); student.setName(name); student.setBranch(branch); student.setPercent(percent); student.setEmail(email); list.add(student); } rs.close(); return list; } }
服务器程序
RMI服务器程序应该实现远程接口或扩展实现类。 在这里,我们应该创建一个远程对象并将其绑定到RMI注册表。
以下是该应用程序的服务器程序。 在这里,我们将扩展上面创建的类,创建一个远程对象并使用绑定名称 hello 将其注册到 RMI 注册表。
import java.rmi.registry.Registry; import java.rmi.registry.LocateRegistry; import java.rmi.RemoteException; import java.rmi.server.UnicastRemoteObject; public class Server extends ImplExample { public Server() {} public static void main(String args[]) { try { // Instantiating the implementation class ImplExample obj = new ImplExample(); // Exporting the object of implementation class ( here we are exporting the remote object to the stub) Hello stub = (Hello) UnicastRemoteObject.exportObject(obj, 0); // Binding the remote object (stub) in the registry Registry registry = LocateRegistry.getRegistry(); registry.bind("Hello", stub); System.err.println("Server ready"); } catch (Exception e) { System.err.println("Server exception: " + e.toString()); e.printStackTrace(); } } }
客户端程序
以下是该应用程序的客户端程序。 在这里,我们获取远程对象并调用名为 getStudents() 的方法。 它从列表对象中检索表的记录并显示它们。
import java.rmi.registry.LocateRegistry; import java.rmi.registry.Registry; import java.util.*; public class Client { private Client() {} public static void main(String[] args)throws Exception { try { // Getting the registry Registry registry = LocateRegistry.getRegistry(null); // Looking up the registry for the remote object Hello stub = (Hello) registry.lookup("Hello"); // Calling the remote method using the obtained object List<Student> list = (List)stub.getStudents(); for (Student s:list)v { // System.out.println("bc "+s.getBranch()); System.out.println("ID: " + s.getId()); System.out.println("name: " + s.getName()); System.out.println("branch: " + s.getBranch()); System.out.println("percent: " + s.getPercent()); System.out.println("email: " + s.getEmail()); } // System.out.println(list); } catch (Exception e) { System.err.println("Client exception: " + e.toString()); e.printStackTrace(); } } }
运行示例的步骤
以下是运行 RMI 示例的步骤。
步骤 1 − 打开存储所有程序的文件夹并编译所有 Java 文件,如下所示。
Javac *.java
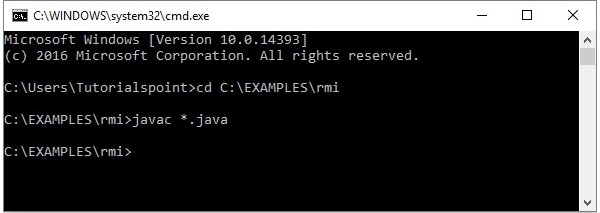
第 2 步 − 使用以下命令启动 rmi 注册表。
start rmiregistry
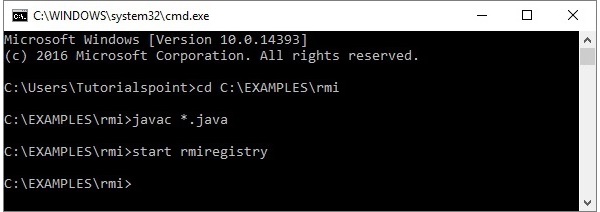
这将在单独的窗口上启动 rmi 注册表,如下所示。
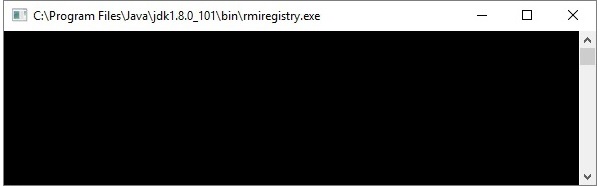
第 3 步 − 运行服务器类文件,如下所示。
Java Server
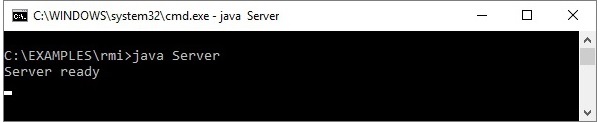
第 4 步 − 运行客户端类文件,如下所示。
java Client
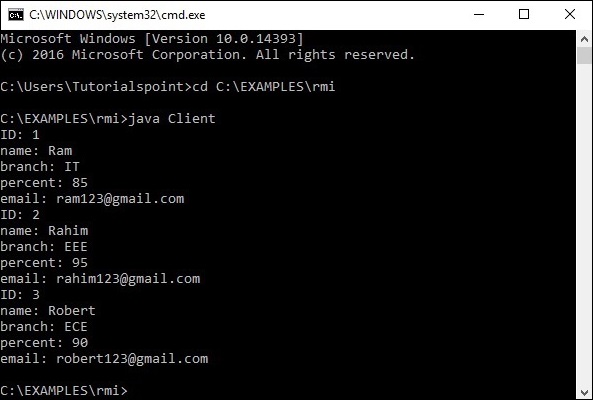