D 语言 if...else 语句
if 语句后面可以跟一个可选的 else 语句,该语句在布尔表达式为 false 时执行。
语法
D 编程语言中 if...else 语句的语法为 −
if(boolean_expression) { /* 如果布尔表达式为 true,则语句将执行 */ } else { /* 如果布尔表达式为 false,则语句将执行 */ }
如果布尔表达式的计算结果为true,则执行if 代码块,否则执行else 代码块。< /p>
D 编程语言将任何非零和非空值假定为true,并且如果它是零 或 null,则假定为 false 值。
流程图
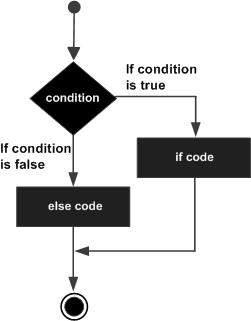
示例
import std.stdio; int main () { /* 局部变量定义 */ int a = 100; /* 检查布尔条件 */ if( a < 20 ) { /* 如果条件为 true 则打印以下内容 */ writefln("a is less than 20" ); } else { /* 如果条件为 false 则打印以下内容 */ writefln("a is not less than 20" ); } writefln("value of a is : %d", a); return 0; }
当上面的代码被编译并执行时,会产生以下结果 −
a is not less than 20; value of a is : 100
if...else if...else 语句
if 语句后面可以跟一个可选的 else if...else 语句,这对于使用单个 if...else if 测试各种条件非常有用 声明。
使用 if、else if、else 语句时有几点需要牢记 −
if 可以有零个或一个 else if,并且它必须位于任何 else if 之后。
if 可以有零到多个 else if,并且它们必须位于 else 之前。
一旦 else if 成功,则不会测试其余的 else if 或 else。
语法
D 编程语言中 if...else if...else 语句的语法为 −
if(boolean_expression 1) { /* 当布尔表达式 1 为 true 时执行 */ } else if( boolean_expression 2) { /* 当布尔表达式 2 为 true 时执行 */ } else if( boolean_expression 3) { /* 当布尔表达式 3 为 true 时执行 */ } else { /* 当上述条件都不成立时执行 */ }
示例
import std.stdio; int main () { /* 局部变量定义 */ int a = 100; /* 检查布尔条件 */ if( a == 10 ) { /* 如果条件为 true 则打印以下内容 */ writefln("Value of a is 10" ); } else if( a == 20 ) { /* if else if 条件为 true */ writefln("Value of a is 20" ); } else if( a == 30 ) { /* if else if 条件为 true */ writefln("Value of a is 30" ); } else { /* 如果没有一个条件为 true */ writefln("None of the values is matching" ); } writefln("Exact value of a is: %d", a ); return 0; }
当上面的代码被编译并执行时,会产生以下结果 −
None of the values is matching Exact value of a is: 100
❮ d_programming_decisions.html