VB.Net - If...Then...Else 语句
If 语句后面可以跟一个可选的 Else 语句,该语句在布尔表达式为 false 时执行。
语法
VB.Net 中 If...Then... Else 语句的语法如下 −
If(boolean_expression)Then '如果布尔表达式为 true,则语句将执行 Else '如果布尔表达式为 false,则语句将执行 End If
如果布尔表达式的计算结果为true,则将执行 if 代码块,否则将执行 else 代码块。
流程图
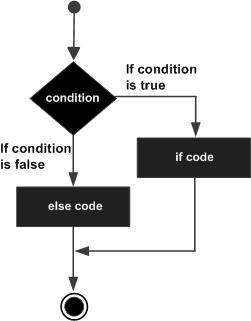
示例
Module decisions Sub Main() '局部变量定义 ' Dim a As Integer = 100 ' 使用 if 语句检查布尔条件 If (a < 20) Then ' 如果条件为 true 则打印以下内容 Console.WriteLine("a is less than 20") Else ' 如果条件为 false 则打印以下内容 Console.WriteLine("a is not less than 20") End If Console.WriteLine("value of a is : {0}", a) Console.ReadLine() End Sub End Module
当上面的代码被编译并执行时,会产生以下结果 −
a is not less than 20 value of a is : 100
If...Else If...Else 语句
If 语句后面可以跟一个可选的 Else if...Else 语句,这对于使用单个 If...Else If 语句测试各种条件非常有用。
使用 If...Else If...Else 语句时,有几点需要记住。
一个 If 可以有零个或一个 Else,并且它必须位于 Else If 之后。
一个 If 可以有零到多个 Else If,并且它们必须位于 Else 之前。
一旦 Else if 成功,则不会测试其余的 Else If 或 Else。
语法
VB.Net 中 if...else if...else 语句的语法如下 −
If(boolean_expression 1)Then ' 当布尔表达式 1 为 true 时执行 ElseIf( boolean_expression 2)Then ' 当布尔表达式 2 为 true 时执行 ElseIf( boolean_expression 3)Then ' 当布尔表达式 3 为 true 时执行 Else ' 当上述条件都不成立时执行 End If
示例
Module decisions Sub Main() '局部变量定义 ' Dim a As Integer = 100 ' 检查布尔条件 ' If (a = 10) Then ' 如果条件为 true 则打印以下内容 ' Console.WriteLine("Value of a is 10") ' ElseIf (a = 20) Then 'if else if 条件为 true ' Console.WriteLine("Value of a is 20") ' ElseIf (a = 30) Then 'if else if 条件为 true Console.WriteLine("Value of a is 30") Else '如果没有一个条件为 true Console.WriteLine("None of the values is matching") End If Console.WriteLine("Exact value of a is: {0}", a) Console.ReadLine() End Sub End Module
当上面的代码被编译并执行时,会产生以下结果 −
None of the values is matching Exact value of a is: 100