Socket.IO - 事件处理
套接字基于事件工作。 有一些保留的事件,可以使用服务器端的套接字对象来访问。
这些是 −
- Connect
- Message
- Disconnect
- Reconnect
- Ping
- Join and
- Leave.
客户端 socket 对象还为我们提供了一些保留事件,这些事件是 −
- Connect
- Connect_error
- Connect_timeout
- Reconnect, etc.
现在,让我们看一个使用 SocketIO 库处理事件的示例。
示例 1
In the Hello World example, we used the connection and disconnection events to log when a user connected and left. Now we will be using the message event to pass message from the server to the client. To do this, modify the io.on ('connection', function(socket)) as shown below –var app = require('express')(); var http = require('http').Server(app); var io = require('socket.io')(http); app.get('/', function(req, res){ res.sendFile('E:/test/index.html'); }); io.on('connection', function(socket){ console.log('A user connected'); // Send a message after a timeout of 4seconds setTimeout(function(){ socket.send('Sent a message 4seconds after connection!'); }, 4000); socket.on('disconnect', function () { console.log('A user disconnected'); }); }); http.listen(3000, function(){ console.log('listening on *:3000'); });
这将在客户端连接后四秒向我们的客户端发送一个名为消息(内置)的事件。 套接字对象上的发送函数关联 'message' 事件。
现在,我们需要在客户端处理此事件,为此,请将index.html页面的内容替换为以下内容 −
<!DOCTYPE html> <html> <head><title>Hello world</title></head> <script src="/socket.io/socket.io.js"></script> <script> var socket = io(); socket.on('message', function(data){document.write(data)}); </script> <body>Hello world</body> </html>
我们现在正在客户端处理 'message' 事件。 当您现在在浏览器中转到该页面时,您将看到以下屏幕截图。
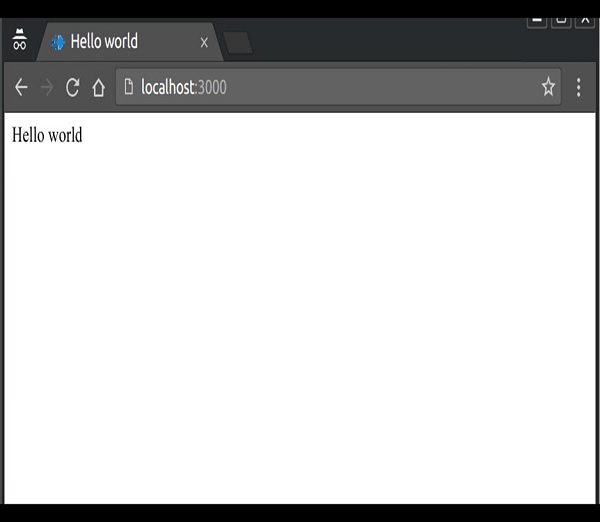
4秒后,服务器发送消息事件,我们的客户端将处理它并产生以下输出 −
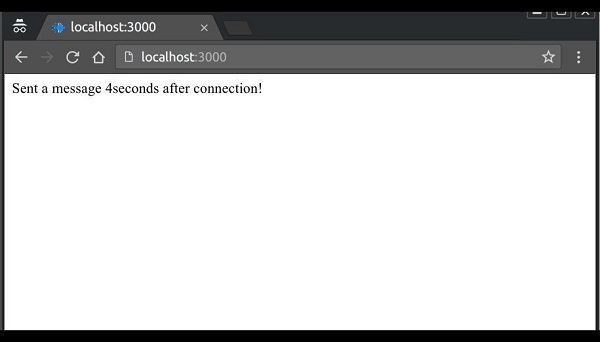
注意 − 我们在此处发送了一串文本; 我们还可以在任何事件中发送一个对象。
Message 是 API 提供的内置事件,但在实际应用中没有多大用处,因为我们需要能够区分事件。
为了实现这一点,Socket.IO 为我们提供了创建自定义事件的能力。 您可以使用 socket.emit 函数创建和触发自定义事件。 以下代码发出一个名为 testerEvent 的事件 −
var app = require('express')(); var http = require('http').Server(app); var io = require('socket.io')(http); app.get('/', function(req, res){ res.sendFile('E:/test/index.html'); }); io.on('connection', function(socket){ console.log('A user connected'); // Send a message when setTimeout(function(){ // Sending an object when emmiting an event socket.emit('testerEvent', { description: 'A custom event named testerEvent!'}); }, 4000); socket.on('disconnect', function () { console.log('A user disconnected'); }); }); http.listen(3000, function(){ console.log('listening on localhost:3000'); });
为了在客户端处理此自定义事件,我们需要一个侦听事件 testerEvent 的侦听器。 以下代码在客户端处理此事件 −
<!DOCTYPE html> <html> <head><title>Hello world</title></head> <script src="/socket.io/socket.io.js"></script> <script> var socket = io(); socket.on('testerEvent', function(data){document.write(data.description)}); </script> <body>Hello world</body> </html>
这将与我们之前的示例以相同的方式工作,在本例中事件是 testerEvent。 当您打开浏览器并访问 localhost:3000 时,您会看到−
Hello world
四秒后,将触发此事件,浏览器会将文本更改为−
A custom event named testerEvent!
示例 2
我们还可以从客户端发出事件。 要从客户端发出事件,请在套接字对象上使用发出函数。
<!DOCTYPE html> <html> <head><title>Hello world</title></head> <script src="/socket.io/socket.io.js"></script> <script> var socket = io(); socket.emit('clientEvent', 'Sent an event from the client!'); </script> <body>Hello world</body> </html>
要处理这些事件,请在服务器上的套接字对象上使用on 函数。
var app = require('express')(); var http = require('http').Server(app); var io = require('socket.io')(http); app.get('/', function(req, res){ res.sendFile('E:/test/index.html'); }); io.on('connection', function(socket){ socket.on('clientEvent', function(data){ console.log(data); }); }); http.listen(3000, function(){ console.log('listening on localhost:3000'); });
所以,现在如果我们访问 localhost:3000,我们将触发一个名为 clientEvent 的自定义事件。 该事件将通过日志记录在服务器上处理 −
Sent an event from the client!