gRPC - 安装和环境设置
Protoc 设置
请注意,只有 Python 才需要设置。对于 Java,所有这些都由 Maven 文件处理。让我们安装"proto"二进制文件,我们将使用它来自动生成".proto"文件的代码。二进制文件可以在 https://github.com/protocolbuffers/protobuf/releases/ 找到。根据操作系统选择正确的二进制文件。我们将在 Windows 上安装 proto 二进制文件,但对于 Linux,步骤没有太大不同。
安装后,请确保您能够通过命令行访问它 −
protoc --version libprotoc 3.15.6
这意味着 Protobuf 已正确安装。现在,让我们转到项目结构。
我们还需要设置 gRPC 代码生成所需的插件。
对于 Python,我们需要执行以下命令 −
python -m pip install grpcio python -m pip install grpcio-tools
它将安装所有必需的二进制文件并将它们添加到路径中。
项目结构
这是我们将拥有的整体项目结构 −

与各个语言相关的代码进入各自的目录。我们将有一个单独的目录来存储我们的原型文件。以下是我们将要使用的 Java 项目结构 −
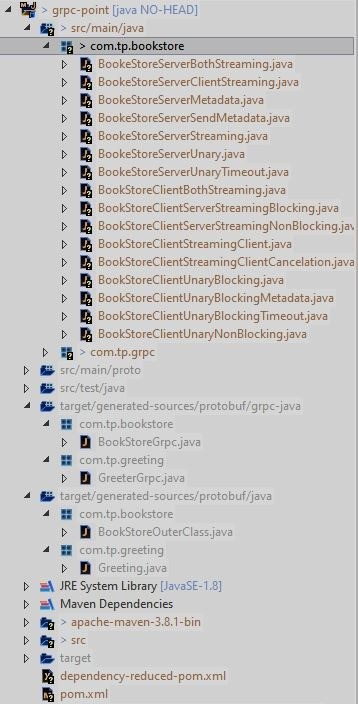
项目依赖关系
现在我们已经安装了 protoc,我们可以使用 protoc 从 proto 文件自动生成代码。让我们首先创建一个 Java 项目。
以下是我们将用于 Java 项目的 Maven 配置。请注意,它还包含 Protobuf 所需的库。
示例
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.grpc.point</groupId> <artifactId>grpc-point</artifactId> <version>1.0</version> <packaging>jar</packaging> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>io.grpc</groupId> <artifactId>grpc-netty-shaded</artifactId> <version>1.38.0</version> </dependency> <dependency> <groupId>io.grpc</groupId> <artifactId>grpc-protobuf</artifactId> <version>1.38.0</version> </dependency> <dependency> <groupId>io.grpc</groupId> <artifactId>grpc-stub</artifactId> <version>1.38.0</version> </dependency> <dependency> <!-- necessary for Java 9+ --> <groupId>org.apache.tomcat</groupId> <artifactId>annotations-api</artifactId> <version>6.0.53</version> <scope>provided</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.slf4j/slf4jsimple--> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.30</version> </dependency> </dependencies> <build> <extensions> <extension> <groupId>kr.motd.maven</groupId> <artifactId>os-maven-plugin</artifactId> <version>1.6.2</version> </extension> </extensions> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>build-helper-maven-plugin</artifactId> <version>1.1</version> <executions> <execution> <id>test</id> <phase>generate-sources</phase> <goals> <goal>add-source</goal> </goals> <configuration> <sources> <source>${basedir}/target/generated-sources</source> </sources> </configuration> </execution> </executions> </plugin> <plugin> <groupId>org.xolstice.maven.plugins</groupId> <artifactId>protobuf-maven-plugin</artifactId> <version>0.6.1</version> <configuration> <protocArtifact>com.google.protobuf:protoc:3.12.0:exe:${os.detected.classifier}</protocArtifact> <pluginId>grpc-java</pluginId> <pluginArtifact>io.grpc:protoc-gen-grpcjava:1.38.0:exe:${os.detected.classifier}</pluginArtifact> <protoSourceRoot>../common_proto_files</protoSourceRoot> </configuration> <executions> <execution> <goals> <goal>compile</goal> <goal>compile-custom</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <version>3.2.4</version> <configuration> </configuration> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> </execution> </executions> </plugin> </plugins> </build> </project>