FastAPI - 参数验证
可以将验证条件应用于路径参数以及 URL 的查询参数。 为了在路径参数上应用验证条件,您需要导入 Path 类。 除了参数的默认值外,对于字符串参数,您还可以指定最大和最小长度。
from fastapi import FastAPI, Path app = FastAPI() @app.get("/hello/{name}") async def hello(name:str=Path(...,min_length=3, max_length=10)): return {"name": name}
如果浏览器的URL中包含长度小于3或大于10的参数,如(http://localhost:8000/hello/Tutorialspoint),则会出现相应的错误信息,如 −
{ "detail": [ { "loc": [ "path", "name" ], "msg": "ensure this value has at most 10 characters", "type": "value_error.any_str.max_length", "ctx": { "limit_value": 10 } } ] }
OpenAPI 文档还显示了应用的验证 −
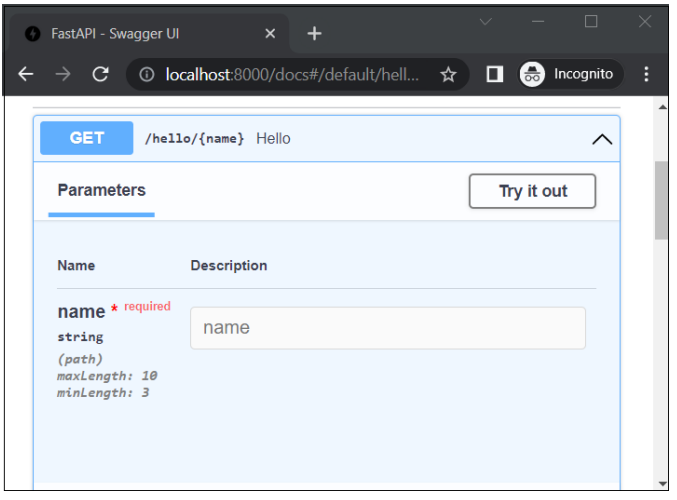
验证规则也可以应用于数字参数,使用下面给出的运算符 −
gt − 大于
ge − 大于等于
lt − 小于
le − 小于等于
让我们修改上面的操作装饰器,将 age 年龄作为路径参数并应用验证。
from fastapi import FastAPI, Path app = FastAPI() @app.get("/hello/{name}/{age}") async def hello(*, name: str=Path(...,min_length=3 , max_length=10), age: int = Path(..., ge=1, le=100)): return {"name": name, "age":age}
在这种情况下,验证规则适用于参数 name 和 age。 如果输入的 URL 为 http://localhost:8000/hello/hi/110,则 JSON 响应显示以下验证失败的解释 −
{ "detail": [ { "loc": [ "path", "name" ], "msg": "ensure this value has at least 3 characters", "type": "value_error.any_str.min_length", "ctx": { "limit_value": 3 } }, { "loc": [ "path", "age" ], "msg": "ensure this value is less than or equal to 100", "type": "value_error.number.not_le", "ctx": { "limit_value": 100 } } ] }
swagger UI 文档还标识了约束。
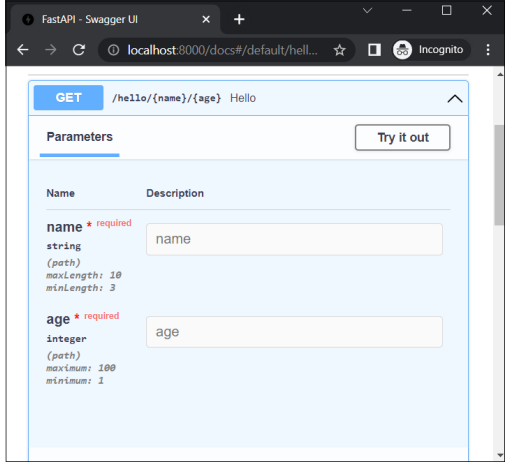
查询参数也可以应用验证规则。 您必须将它们指定为 Query 类构造函数的参数的一部分。
让我们在上面的函数中添加一个名为 percent 的查询参数,并将验证规则应用为 ge=0(即大于等于 0)和 lt=100(小于等于100)
from fastapi import FastAPI, Path, Query @app.get("/hello/{name}/{age}") async def hello(*, name: str=Path(...,min_length=3 , max_length=10), \ age: int = Path(..., ge=1, le=100), \ percent:float=Query(..., ge=0, le=100)): return {"name": name, "age":age}
如果输入的 URL 是 http://localhost:8000/hello/Ravi/20?percent=79,那么浏览器会显示如下的 JSON 响应 −
{"name":"Ravi","age":20}
FastAPI 在应用了验证条件的情况下正确地将 percent 识别为查询参数。 在 OpenAPI 文档中体现如下 −
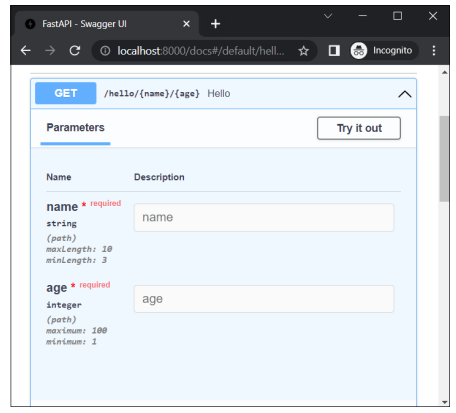
虽然客户端可以使用 GET 方法向 API 服务器发送路径和查询参数,但我们需要应用 POST 方法将一些二进制数据作为 HTTP 请求的一部分发送。 此二进制数据可以是任何 Python 类的对象形式。 它构成请求主体。 为此,FastAPI 使用 Pydantic 库。