SWING - GroupLayout 类
简介
GroupLayout 类对组件进行分层分组,以便将它们放置在容器中。
类声明
以下是 javax.swing.GroupLayout 类的声明 −
public class GroupLayout extends Object implements LayoutManager2
字段
以下是 javax.swing.GroupLayout 类的字段 −
static int DEFAULT_SIZE − 指示组件的大小或间隙应用于特定范围值。
static int PREFERRED_SIZE − 指示组件的首选大小或间隙应用于特定范围值。
类构造函数
序号 | 构造函数 & 描述 |
---|---|
1 |
GroupLayout(Container host) 为指定的 Container 创建一个 GroupLayout。 |
类方法
序号 | 方法 & 描述 |
---|---|
1 |
void addLayoutComponent(Component component, Object constraints) 组件已添加到父容器的通知。 |
2 |
void addLayoutComponent(String name, Component component) 组件已添加到父容器的通知。 |
3 |
GroupLayout.ParallelGroup createBaselineGroup(boolean resizable, boolean anchorBaselineToTop) 创建并返回将其元素沿基线对齐的并行组。 |
4 |
GroupLayout.ParallelGroup createParallelGroup() 创建并返回对齐方式为 Alignment.LEADING 的 ParallelGroup。 |
5 |
GroupLayout.ParallelGroup createParallelGroup(GroupLayout.Alignment alignment) 创建并返回具有指定对齐方式的 ParallelGroup。 |
6 |
GroupLayout.ParallelGroup createParallelGroup(GroupLayout.Alignment alignment, boolean resizable) 创建并返回具有指定对齐和调整大小行为的 ParallelGroup。 |
7 |
GroupLayout.SequentialGroup createSequentialGroup() 创建并返回一个 SequentialGroup。 |
8 |
boolean getAutoCreateContainerGaps() 如果容器和容器边界的组件之间的间隙是自动创建的,则返回 true。 |
9 |
boolean getAutoCreateGaps() 如果组件之间的间隙是自动创建的,则返回 true。 |
10 |
boolean getHonorsVisibility() 返回在调整组件大小和定位组件时是否考虑组件可见性。 |
11 |
float getLayoutAlignmentX(Container parent) 返回沿 x 轴的对齐方式。 |
12 |
float getLayoutAlignmentY(Container parent) 返回沿 y 轴的对齐方式。 |
13 |
LayoutStyle getLayoutStyle() 返回用于计算组件之间首选间隙的 LayoutStyle。 |
14 |
void invalidateLayout(Container parent) 使布局无效,指示如果布局管理器缓存了信息,则应将其丢弃。 |
15 |
void layoutContainer(Container parent) 布置指定的容器。 |
16 |
void linkSize(Component... components) 强制指定组件具有相同的大小,而不管它们的首选、最小或最大大小。 |
17 |
void linkSize(int axis, Component... components) 强制指定组件沿指定轴具有相同的大小,而不管它们的首选、最小或最大尺寸。 |
18 |
Dimension maximumLayoutSize(Container parent) 返回指定容器的最大大小。 |
19 |
Dimension minimumLayoutSize(Container parent) 返回指定容器的最小大小。 |
20 |
Dimension preferredLayoutSize(Container parent) 返回指定容器的首选大小。 |
21 |
void removeLayoutComponent(Component component) 通知组件已从父容器中移除。 |
22 |
void replace(Component existingComponent, Component newComponent) 用新组件替换现有组件。 |
23 |
void setAutoCreateContainerGaps(boolean autoCreateContainerPadding) 设置容器和接触容器边框的组件之间是否应自动创建间隙。 |
24 |
void setAutoCreateGaps(boolean autoCreatePadding) 设置是否应自动创建组件之间的间隙。 |
25 |
void setHonorsVisibility(boolean honorsVisibility) 设置在调整组件大小和定位组件时是否考虑组件可见性。 |
26 |
void setHonorsVisibility(Component component, Boolean honorsVisibility) 设置是否在调整大小和定位时考虑组件可见性。 |
27 |
void setHorizontalGroup(GroupLayout.Group group) 设置沿水平轴定位和调整组件大小的组。 |
28 |
void setLayoutStyle(LayoutStyle layoutStyle) 设置用于计算组件之间首选间隙的 LayoutStyle。 |
29 |
void setVerticalGroup(GroupLayout.Group group) 设置沿垂直轴定位和调整组件大小的组。 |
30 |
String toString() 返回此 GroupLayout 的字符串表示形式。 |
继承的方法
这个类继承了以下类的方法 −
- java.lang.Object
GroupLayout 示例
在 D:/ > SWING > com > tutorialspoint > gui > 中使用您选择的任何编辑器创建以下 Java 程序
SwingLayoutDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import javax.swing.*; public class SwingLayoutDemo { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; private JLabel msglabel; public SwingLayoutDemo(){ prepareGUI(); } public static void main(String[] args){ SwingLayoutDemo swingLayoutDemo = new SwingLayoutDemo(); swingLayoutDemo.showGroupLayoutDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Java SWING Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); headerLabel = new JLabel("",JLabel.CENTER ); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,100); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showGroupLayoutDemo(){ headerLabel.setText("Layout in action: GroupLayout"); JPanel panel = new JPanel(); // panel.setBackground(Color.darkGray); panel.setSize(200,200); GroupLayout layout = new GroupLayout(panel); layout.setAutoCreateGaps(true); layout.setAutoCreateContainerGaps(true); JButton btn1 = new JButton("Button 1"); JButton btn2 = new JButton("Button 2"); JButton btn3 = new JButton("Button 3"); layout.setHorizontalGroup(layout.createSequentialGroup() .addComponent(btn1) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup( GroupLayout.Alignment.LEADING) .addComponent(btn2) .addComponent(btn3)))); layout.setVerticalGroup(layout.createSequentialGroup() .addComponent(btn1) .addComponent(btn2) .addComponent(btn3)); panel.setLayout(layout); controlPanel.add(panel); mainFrame.setVisible(true); } }
使用命令提示符编译程序。 转到 D:/ > SWING 并键入以下命令。
D:\SWING>javac com\tutorialspoint\gui\SwingLayoutDemo.java
如果没有报错,说明编译成功。 使用以下命令运行程序。
D:\SWING>java com.tutorialspoint.gui.SwingLayoutDemo
验证以下输出。
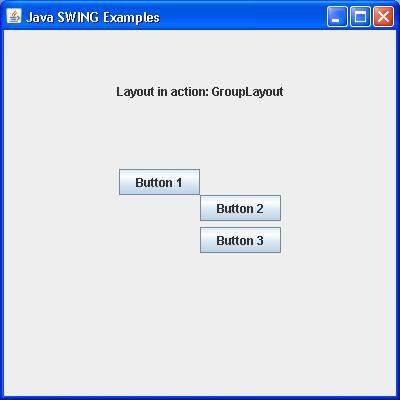