如何使用 Java 从系统相机拍摄快照
问题描述
如何使用 Java 从系统相机拍摄快照。
解决方案
以下是使用 Java 从系统相机拍摄快照的程序。
import java.awt.image.BufferedImage; import java.awt.image.DataBufferByte; import java.awt.image.WritableRaster; import java.io.FileNotFoundException; import java.io.IOException; import javafx.application.Application; import javafx.embed.swing.SwingFXUtils; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.image.ImageView; import javafx.scene.image.WritableImage; import javafx.stage.Stage; import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.videoio.VideoCapture; public class TakingSnapshotUsingCamera extends Application { Mat matrix = null; @Override public void start(Stage stage) throws FileNotFoundException, IOException { //从相机捕获快照 TakingSnapshotUsingCamera obj = new TakingSnapshotUsingCamera(); WritableImage writableImage = obj.capureSnapShot(); //设置图像视图 ImageView imageView = new ImageView(writableImage); //设置图像视图的适合高度和宽度 imageView.setFitHeight(400); imageView.setFitWidth(600); //设置图像视图的保留比例 imageView.setPreserveRatio(true); //创建 Group 对象 Group root = new Group(imageView); //创建场景对象 Scene scene = new Scene(root, 600, 400); //设置stage(舞台)标题 stage.setTitle("Capturing an image"); //将场景添加到stage(舞台) stage.setScene(scene); //显示stage(舞台)内容 stage.show(); } public WritableImage capureSnapShot() { WritableImage WritableImage = null; //加载 OpenCV 核心库 System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); //实例化 VideoCapture 类 (camera:: 0) VideoCapture capture = new VideoCapture(0); //从摄像头读取下一个视频帧 Mat matrix = new Mat(); capture.read(matrix); //如果摄像头已打开 if(capture.isOpened()) { //如果有下一个视频帧 if (capture.read(matrix)) { //从矩阵创建 BuffredImage BufferedImage image = new BufferedImage(matrix.width(), matrix.height(), BufferedImage.TYPE_3BYTE_BGR); WritableRaster raster = image.getRaster(); DataBufferByte dataBuffer = (DataBufferByte) raster.getDataBuffer(); byte[] data = dataBuffer.getData(); matrix.get(0, 0, data); this.matrix = matrix; //创建可写映像 WritableImage = SwingFXUtils.toFXImage(image, null); } } return WritableImage; } public static void main(String args[]) { launch(args); } }
输出
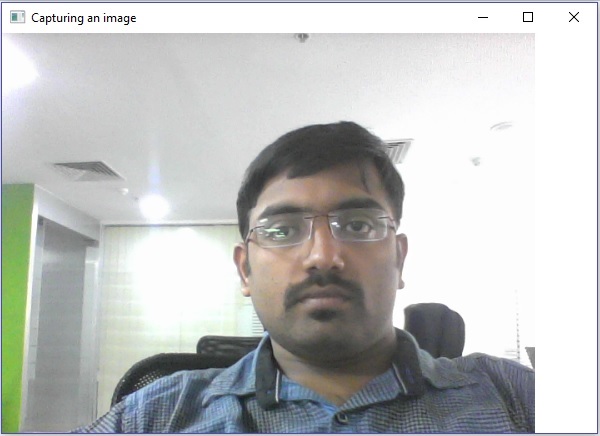
java_opencv.html